21、标准库初探
大约 2 分钟C++C++基础编程程序厨
标准库是C++
的重要组成部分,它提供了非常丰富的能力,包括输入输出操作、字符串处理、容器、算法、数值运算等多个方面。
下面介绍常见的部分。
命名空间std
标准库的组件都放在命名空间std
中,避免命名冲突。
建议在代码中使用std::
前缀,而不是using namespace std;
,尤其是在大型项目中。
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
常用的标准库函数
比如数学函数,<cmath>
头文件提供了许多数学函数。
计算平方根:sqrt
#include <iostream>
#include <cmath>
int main() {
double number = 16.0;
double squareRoot = sqrt(number);
std::cout << "The square root of " << number << " is " << squareRoot << std::endl;
return 0;
}
计算幂:pow
#include <iostream>
#include <cmath>
int main() {
double base = 2.0;
double exponent = 3.0;
double result = pow(base, exponent);
std::cout << base << " raised to the power of " << exponent << " is " << result << std::endl;
return 0;
}
标准输入输出流库(iostream)
标准输入输出流
#include <iostream>
int main() {
int number;
std::cout << "Please enter a number: ";
std::cin >> number;
std::cout << "You entered: " << number << std::endl;
return 0;
}
格式化输入输出
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.14159265;
std::cout << "Pi is approximately " << std::fixed << std::setprecision(2) << pi << std::endl;
return 0;
}
字符串库(string)
创建和初始化字符串
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello, World!";
std::string str2;
str2 = "Hello again!";
std::cout << str1 << std::endl;
std::cout << str2 << std::endl;
return 0;
}
字符串拼接
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello";
std::string name = "Alice";
std::string message = greeting + " " + name + "!";
std::cout << message << std::endl;
return 0;
}
字符串查找和修改
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
size_t found = text.find("World");
if (found != std::string::npos) {
std::cout << "Found 'World' at position: " << found << std::endl;
text.replace(found, 5, "C++");
std::cout << "Modified string: " << text << std::endl;
}
return 0;
}
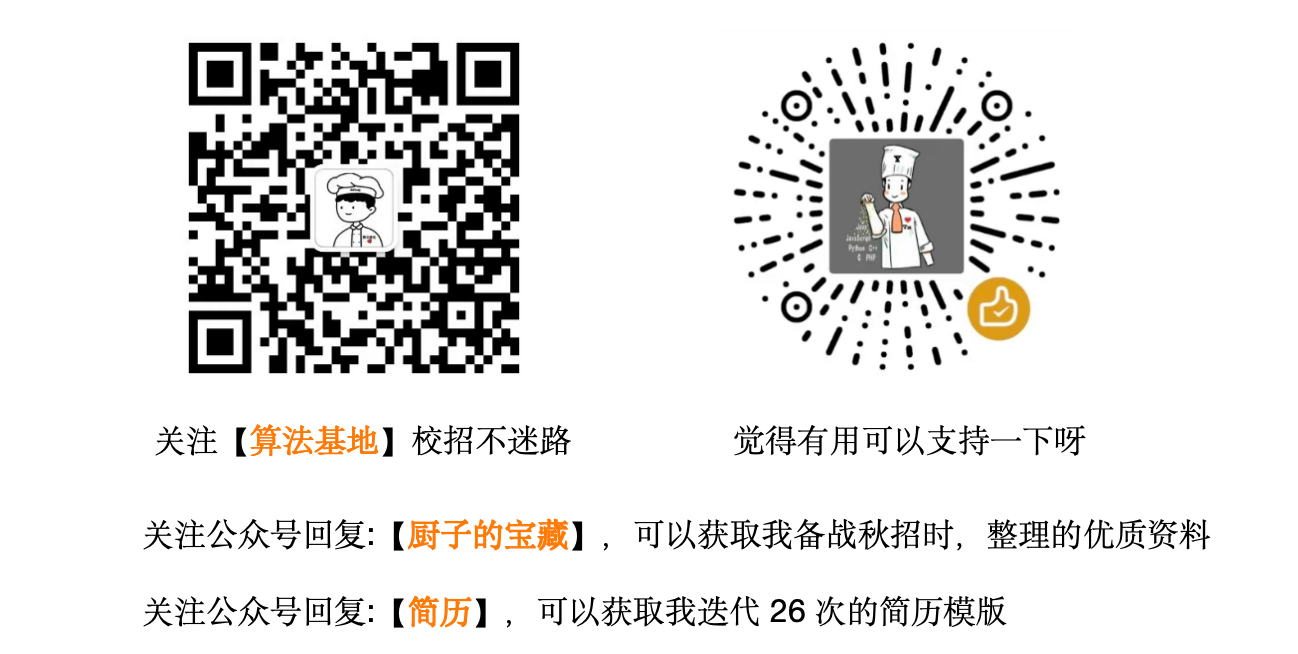