35、C语言中的标准库函数
大约 4 分钟C语言基础程序程序厨
本文主要介绍C语言中一些常见的各个方向的标准库函数。
字符串处理函数
C语言提供了一系列用于处理字符串的函数,这些函数定义在<string.h>
头文件中:
- strcpy(char *dest, const char *src): 将字符串src复制到字符串dest中,包括终止字符'\0'。
- strcat(char *dest, const char *src): 将字符串src连接到字符串dest的末尾。
- strlen(const char *str): 计算字符串str的长度,不包括终止字符'\0'。
- strcmp(const char *str1, const char *str2): 比较字符串str1和str2。如果str1小于、等于或大于str2,则分别返回负整数、零或正整数。
- strchr(const char *str, int c): 在字符串str中查找字符c的第一次出现,并返回一个指向该字符的指针。如果未找到该字符,则返回NULL。
- strstr(const char *str1, const char *str2): 在字符串str1中查找字符串str2的第一次出现,并返回一个指向str1中str2起始位置的指针。如果未找到str2,则返回NULL。
示例代码:
#include <stdio.h>
#include <string.h>
int main() {
char dest[50] = "Hello, ";
const char *src = "World!";
strcpy(dest + strlen(dest), src); // 使用strcpy连接字符串
printf("%s\n", dest); // 输出: Hello, World!
char str[] = "Example String";
char *pos = strchr(str, 'e'); // 查找字符'e'
if (pos != NULL) {
printf("Character 'e' found at position: %ld\n", pos - str);
}
const char *substr = "String";
pos = strstr(str, substr); // 查找子字符串
if (pos != NULL) {
printf("Substring found at position: %ld\n", pos - str);
}
return 0;
}
为了安全,C11标准引入了一组字符串处理函数,比如strncpy
、strncat
、strncmp
等函数,指定要复制或比较的最大字符数,从而防止缓冲区溢出,我们在项目中一定要用这些安全的函数。
数学函数与随机数生成
C语言标准库提供了丰富的数学函数,这些函数定义在<math.h>
头文件中:
- sqrt(double x): 计算x的平方根。
- pow(double base, double exponent): 计算base的exponent次幂。
- sin(double x): 计算x(以弧度为单位)的正弦值。
- cos(double x): 计算x(以弧度为单位)的余弦值。
- fabs(double x): 计算x的绝对值。
随机数生成:
C语言通过<stdlib.h>
头文件中的函数提供随机数生成功能。
- rand(): 返回一个在0到
RAND_MAX
之间的伪随机数。 - srand(unsigned int seed): 使用seed作为随机数生成器的种子。如果不调用srand或使用相同的种子值调用srand,则每次程序运行时rand()将产生相同的序列。
示例代码:
#include <stdio.h>
#include <math.h>
#include <stdlib.h>
#include <time.h>
int main() {
double num = 9.0;
printf("Square root of %.2f is %.2f\n", num, sqrt(num));
printf("2 raised to the power of 3 is %.2f\n", pow(2.0, 3.0));
// 设置随机数种子为当前时间
srand(time(0));
printf("Random number between 0 and RAND_MAX: %d\n", rand());
// 生成0到99之间的随机数
int random_num = rand() % 100;
printf("Random number between 0 and 99: %d\n", random_num);
return 0;
}
日期与时间函数
C语言标准库通过<time.h>
头文件提供了处理日期和时间的函数:
- time(time_t *timer): 获取当前时间(自1970年1月1日以来的秒数)。
- localtime(const time_t *timer): 将time_t类型的时间转换为本地时间(struct tm结构)。
- gmtime(const time_t *timer): 将time_t类型的时间转换为协调世界时(UTC,struct tm结构)。
- strftime(char *str, size_t maxsize, const char *format, const struct tm *timeptr): 根据指定的格式将时间转换为字符串。
示例代码:
#include <stdio.h>
#include <time.h>
int main() {
time_t current_time;
struct tm *local_time_info;
// 获取当前时间
time(¤t_time);
// 转换为本地时间
local_time_info = localtime(¤t_time);
// 打印当前时间
printf("Current local time and date: %s", asctime(local_time_info));
// 使用strftime格式化时间
char buffer[80];
strftime(buffer, sizeof(buffer), "%Y-%m-%d %H:%M:%S", local_time_info);
printf("Formatted date and time: %s\n", buffer);
return 0;
}
对于需要高精度时间的应用程序(如实时系统),可以使用POSIX标准提供的clock_gettime()
函数。
其他常用库函数
C语言标准库还提供了许多其他有用的函数,这些函数通常定义在<stdlib.h>
、<stdio.h>
等头文件中。以下是一些常用的函数:
- malloc(size_t size): 动态分配指定大小的内存块。
- free(void *ptr): 释放之前动态分配的内存块。
- printf(const char *format, ...): 格式化输出到标准输出。
- scanf(const char *format, ...): 从标准输入读取并格式化数据。
- exit(int status): 终止程序并返回状态码。
示例代码:
#include <stdio.h>
#include <stdlib.h>
int main() {
int *arr = (int *)malloc(5 * sizeof(int)); // 动态分配内存
if (arr == NULL) {
perror("Failed to allocate memory");
exit(EXIT_FAILURE);
}
// 使用分配的内存
for (int i = 0; i < 5; i++) {
arr[i] = i * 2;
}
// 打印数组内容
for (int i = 0; i < 5; i++) {
printf("%d ", arr[i]);
}
printf("\n");
// 释放内存
free(arr);
return 0;
}
练习
- 打印当前时间字符串到屏幕中。
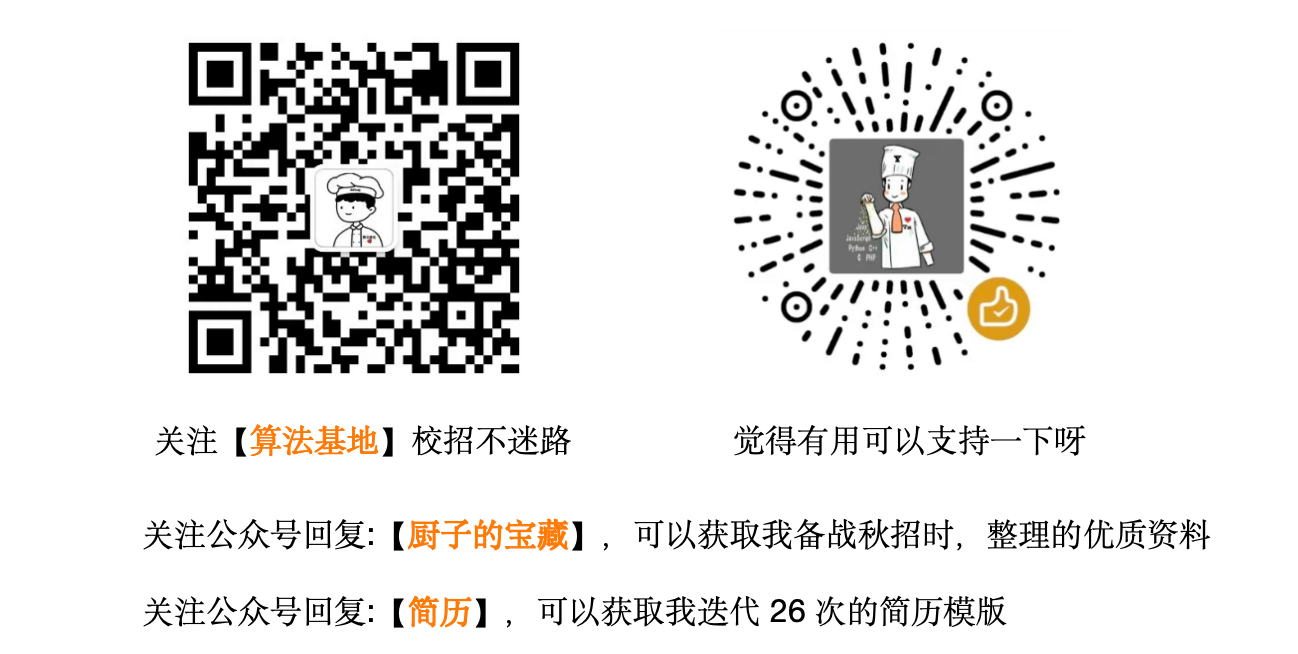